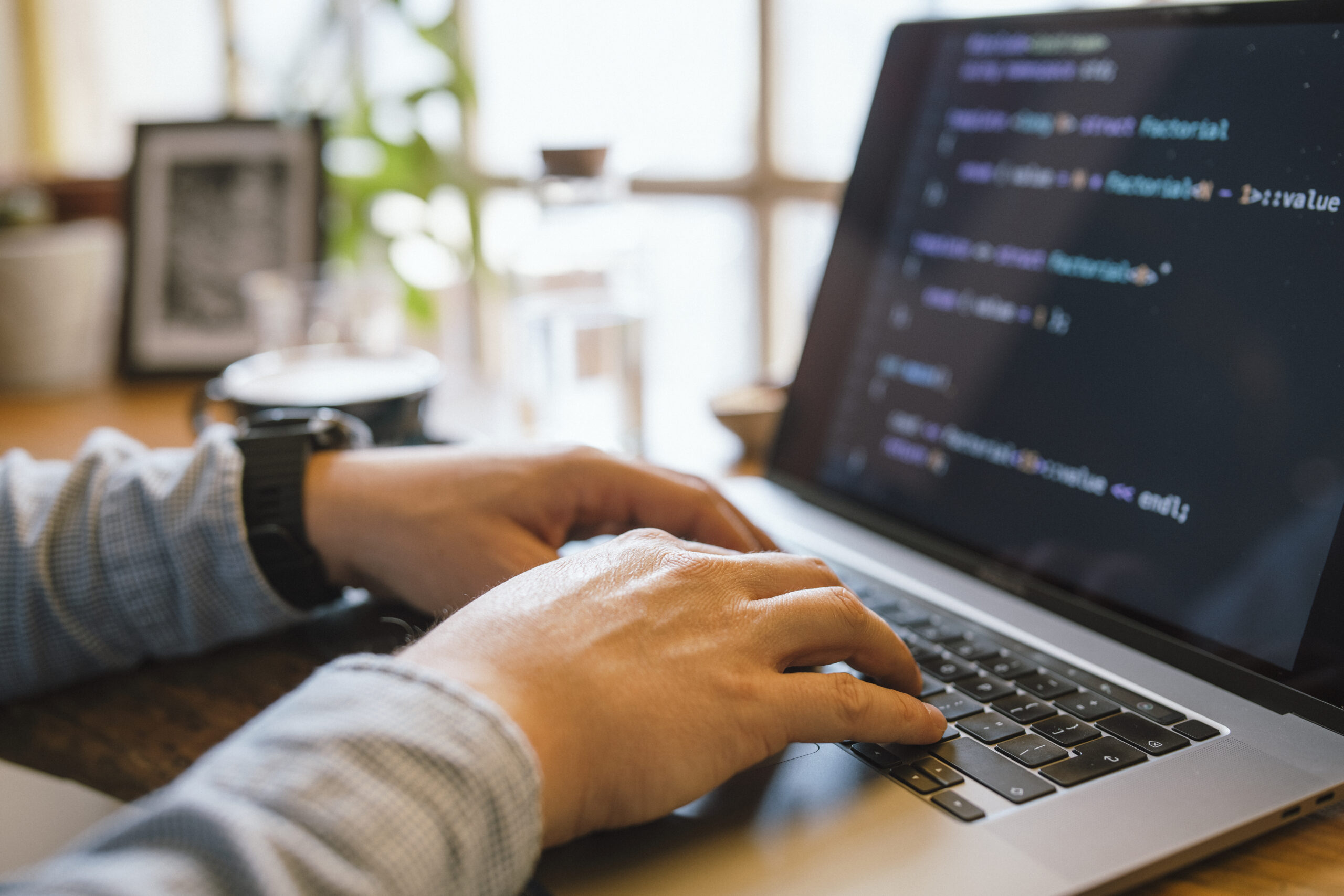
Debugging is Among the most essential — nevertheless generally missed — abilities within a developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about knowledge how and why things go Incorrect, and Finding out to Assume methodically to unravel complications efficiently. Regardless of whether you're a novice or maybe a seasoned developer, sharpening your debugging competencies can help you save several hours of irritation and radically help your efficiency. Here i will discuss quite a few procedures that can help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of many quickest methods developers can elevate their debugging abilities is by mastering the tools they use every day. While crafting code is a person part of development, knowing how to connect with it correctly through execution is Similarly crucial. Contemporary development environments occur Outfitted with effective debugging capabilities — but many builders only scratch the surface area of what these equipment can do.
Consider, for instance, an Built-in Progress Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools allow you to set breakpoints, inspect the worth of variables at runtime, move by way of code line by line, and also modify code on the fly. When made use of effectively, they Allow you to observe particularly how your code behaves for the duration of execution, which can be invaluable for tracking down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-finish developers. They assist you to inspect the DOM, watch community requests, look at actual-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can switch aggravating UI problems into workable duties.
For backend or process-degree builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB offer deep Command in excess of working processes and memory administration. Understanding these tools could possibly have a steeper Studying curve but pays off when debugging general performance challenges, memory leaks, or segmentation faults.
Over and above your IDE or debugger, become relaxed with Variation Handle units like Git to be aware of code historical past, obtain the exact minute bugs ended up introduced, and isolate problematic alterations.
Finally, mastering your resources suggests going over and above default configurations and shortcuts — it’s about developing an intimate familiarity with your enhancement environment in order that when difficulties crop up, you’re not lost at the hours of darkness. The better you already know your tools, the more time you may shell out fixing the actual difficulty as an alternative to fumbling by the procedure.
Reproduce the issue
Just about the most important — and infrequently forgotten — techniques in productive debugging is reproducing the situation. In advance of leaping to the code or producing guesses, developers require to make a constant atmosphere or situation in which the bug reliably appears. With out reproducibility, correcting a bug results in being a activity of probability, generally resulting in wasted time and fragile code adjustments.
The first step in reproducing a dilemma is accumulating as much context as possible. Talk to issues like: What actions led to The difficulty? Which setting was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you may have, the much easier it becomes to isolate the precise conditions less than which the bug happens.
Once you’ve gathered sufficient information and facts, try and recreate the issue in your neighborhood atmosphere. This may imply inputting a similar knowledge, simulating comparable consumer interactions, or mimicking system states. If The problem appears intermittently, look at creating automatic tests that replicate the edge conditions or state transitions included. These tests not just enable expose the issue and also avoid regressions Sooner or later.
Occasionally, The problem may very well be atmosphere-precise — it'd occur only on selected functioning methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms might be instrumental in replicating this kind of bugs.
Reproducing the situation isn’t just a action — it’s a state of mind. It necessitates tolerance, observation, and a methodical strategy. But as soon as you can consistently recreate the bug, you are by now midway to fixing it. By using a reproducible state of affairs, You can utilize your debugging instruments much more efficiently, examination probable fixes safely and securely, and converse additional Evidently with all your group or consumers. It turns an abstract complaint into a concrete challenge — and that’s exactly where developers prosper.
Read and Understand the Mistake Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Erroneous. In lieu of looking at them as frustrating interruptions, builders should master to take care of mistake messages as direct communications from the system. They normally show you just what exactly took place, in which it happened, and sometimes even why it transpired — if you understand how to interpret them.
Commence by reading the information meticulously and in full. Lots of builders, particularly when under time tension, glance at the very first line and instantly begin making assumptions. But further while in the error stack or logs might lie the legitimate root bring about. Don’t just copy and paste mistake messages into serps — read through and realize them first.
Split the error down into sections. Is it a syntax mistake, a runtime exception, or even a logic mistake? Does it point to a certain file and line quantity? What module or functionality activated it? These questions can information your investigation and stage you towards the responsible code.
It’s also beneficial to understand the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java often follow predictable designs, and learning to recognize these can greatly accelerate your debugging course of action.
Some mistakes are obscure or generic, As well as in those circumstances, it’s very important to examine the context during which the mistake happened. Verify connected log entries, enter values, and up to date modifications while in the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede much larger issues and provide hints about prospective bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and become a more effective and self-confident developer.
Use Logging Sensibly
Logging is Probably the most effective equipment in a very developer’s debugging toolkit. When made use of effectively, it provides genuine-time insights into how an application behaves, aiding you realize what’s occurring beneath the hood while not having to pause execution or action from the code line by line.
A very good logging system starts off with figuring out what to log and at what stage. Popular logging concentrations consist of DEBUG, Information, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic information throughout advancement, Details for normal situations (like successful begin-ups), WARN for possible problems that don’t break the applying, Mistake for true difficulties, and FATAL when the procedure can’t keep on.
Keep away from flooding your logs with extreme or irrelevant data. Excessive logging can obscure crucial messages and slow down your process. Target crucial events, point out adjustments, input/output values, and significant selection details as part of your code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace problems in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
During debugging, logs Permit you to keep track of how variables evolve, what circumstances are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Specifically valuable in generation environments exactly where stepping by code isn’t probable.
Furthermore, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about stability and clarity. With a properly-assumed-out logging strategy, you could reduce the time it's going to take to spot troubles, gain deeper visibility into your apps, and Increase the All round maintainability and trustworthiness of click here your code.
Consider Similar to a Detective
Debugging is not just a specialized process—it is a form of investigation. To efficiently establish and fix bugs, developers have to solution the process like a detective fixing a thriller. This mentality assists break down sophisticated difficulties into manageable components and comply with clues logically to uncover the basis bring about.
Get started by gathering evidence. Look at the signs of the challenge: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, gather as much related details as you'll be able to with no jumping to conclusions. Use logs, examination situations, and consumer studies to piece collectively a clear image of what’s happening.
Subsequent, form hypotheses. Ask yourself: What could be causing this actions? Have any variations a short while ago been built into the codebase? Has this challenge transpired ahead of beneath equivalent conditions? The aim would be to narrow down possibilities and detect probable culprits.
Then, take a look at your theories systematically. Try and recreate the trouble in a managed setting. Should you suspect a specific functionality or part, isolate it and verify if the issue persists. Similar to a detective conducting interviews, question your code queries and let the final results direct you nearer to the reality.
Spend shut consideration to little aspects. Bugs generally conceal during the minimum expected spots—like a lacking semicolon, an off-by-1 mistake, or possibly a race situation. Be thorough and client, resisting the urge to patch the issue without absolutely knowledge it. Short-term fixes may well conceal the actual issue, just for it to resurface afterwards.
And finally, keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging method can help you save time for long term problems and support others recognize your reasoning.
By wondering like a detective, builders can sharpen their analytical competencies, method troubles methodically, and become more effective at uncovering hidden difficulties in complex techniques.
Produce Checks
Writing exams is among the simplest ways to increase your debugging techniques and In general improvement effectiveness. Assessments don't just help catch bugs early but additionally serve as a safety Internet that provides you self esteem when earning changes for your codebase. A effectively-examined application is simpler to debug since it lets you pinpoint particularly wherever and when a dilemma takes place.
Get started with device assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can promptly expose irrespective of whether a selected bit of logic is Performing as predicted. Every time a examination fails, you quickly know the place to search, substantially lowering the time spent debugging. Device assessments are Specially beneficial for catching regression bugs—troubles that reappear right after previously being preset.
Upcoming, integrate integration tests and close-to-conclusion assessments into your workflow. These aid make sure that various aspects of your software perform together effortlessly. They’re notably beneficial for catching bugs that occur in advanced programs with numerous factors or companies interacting. If one thing breaks, your checks can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to definitely think critically regarding your code. To test a element correctly, you need to be aware of its inputs, expected outputs, and edge scenarios. This degree of knowledge By natural means potential customers to better code composition and fewer bugs.
When debugging a concern, creating a failing test that reproduces the bug might be a robust first step. When the test fails persistently, you can target correcting the bug and observe your take a look at pass when the issue is solved. This solution ensures that the exact same bug doesn’t return Down the road.
In brief, composing checks turns debugging from the aggravating guessing video game right into a structured and predictable process—aiding you capture extra bugs, faster and even more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the situation—gazing your monitor for hours, attempting Remedy immediately after Alternative. But one of the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lower annoyance, and infrequently see The difficulty from the new standpoint.
If you're much too near the code for much too long, cognitive fatigue sets in. You might start overlooking obvious errors or misreading code that you wrote just hours before. In this point out, your Mind results in being fewer economical at challenge-fixing. A short walk, a coffee crack, or maybe switching to a unique process for 10–15 minutes can refresh your focus. Many builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, permitting their subconscious operate inside the background.
Breaks also help reduce burnout, In particular for the duration of for a longer time debugging sessions. Sitting before a display screen, mentally stuck, is not only unproductive but will also draining. Stepping absent means that you can return with renewed Electricity plus a clearer state of mind. You might quickly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you ahead of.
In the event you’re stuck, a very good guideline is always to established a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that time to maneuver around, extend, or do one thing unrelated to code. It may well sense counterintuitive, Specifically less than restricted deadlines, but it really in fact leads to a lot quicker and more effective debugging Ultimately.
Briefly, taking breaks is just not an indication of weakness—it’s a smart tactic. It gives your brain Place to breathe, improves your point of view, and allows you stay away from the tunnel eyesight that often blocks your progress. Debugging is usually a mental puzzle, and relaxation is part of fixing it.
Learn From Each and every Bug
Each individual bug you experience is much more than simply A short lived setback—It is a chance to mature as a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can train you a little something beneficial should you take the time to reflect and evaluate what went Mistaken.
Start out by inquiring yourself a couple of important queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like unit testing, code reviews, or logging? The answers often reveal blind places in your workflow or comprehending and assist you to Construct more powerful coding practices relocating forward.
Documenting bugs can be a superb routine. Preserve a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll start to see patterns—recurring challenges or popular faults—you can proactively prevent.
In crew environments, sharing Whatever you've discovered from a bug with all your friends might be Specifically effective. Whether or not it’s via a Slack information, a short write-up, or A fast expertise-sharing session, assisting others steer clear of the identical issue boosts staff effectiveness and cultivates a stronger Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your state of mind from irritation to curiosity. In place of dreading bugs, you’ll commence appreciating them as essential portions of your advancement journey. After all, several of the very best builders aren't those who create great code, but those that consistently discover from their faults.
Ultimately, Just about every bug you resolve provides a brand new layer on your skill set. So future time you squash a bug, take a minute to replicate—you’ll come absent a smarter, extra capable developer as a consequence of it.
Summary
Bettering your debugging techniques requires time, follow, and tolerance — however the payoff is big. It would make you a more effective, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.